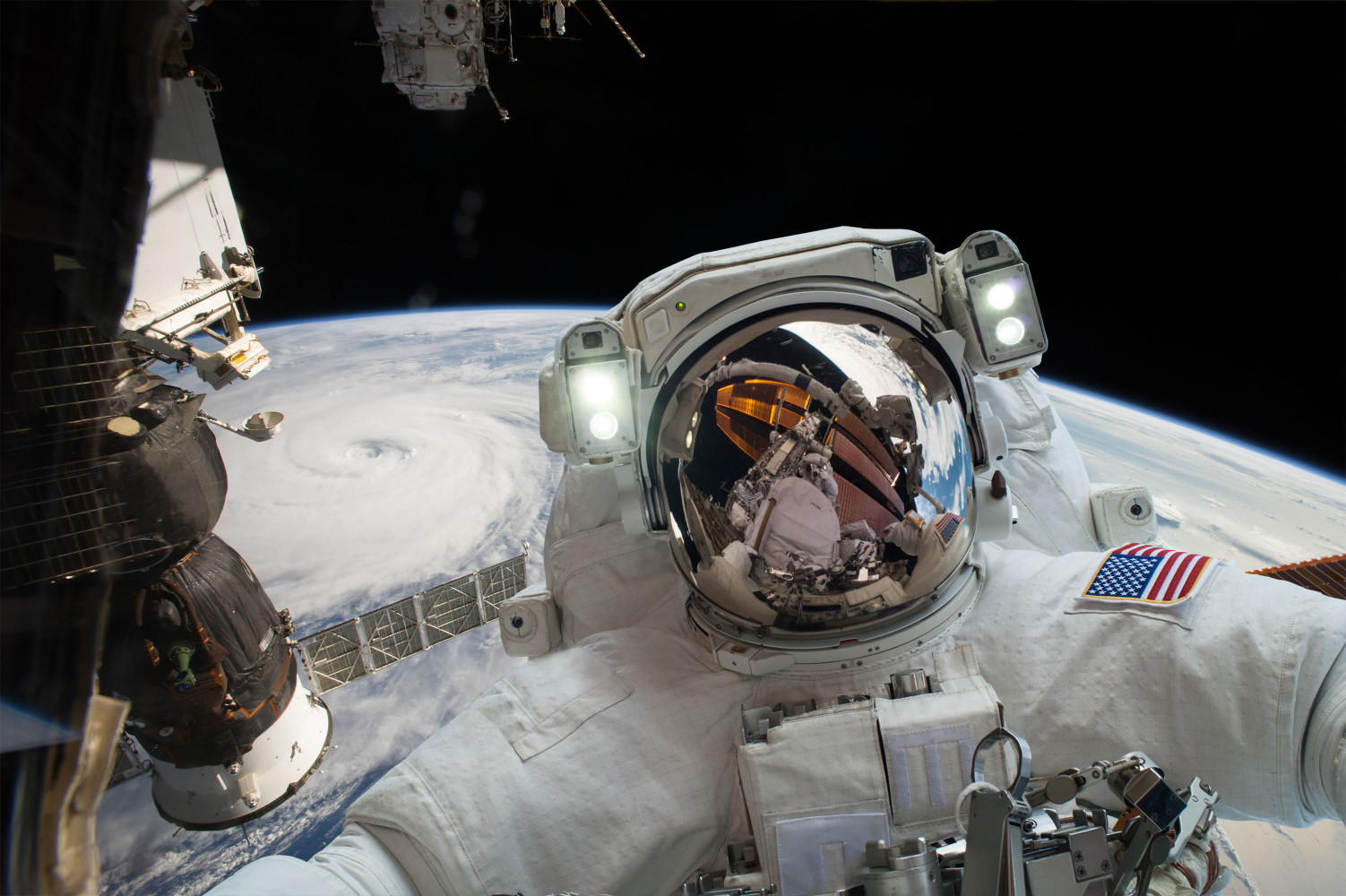
Making the case for TypeScript — or why and how you can bring your frontend development to the next level and do yourself and the world something good.
We’ve all been there: You fire up a browser to get a preview of the application you are developing — and it doesn’t work. Plus, it’s not just some slightly buggy behaviour of a corner case scenario, not at all. You just get a blank screen: “what a lovely white canvas we got here”, immediately goes through your head, “not that it hasn't ever happened before”. After yet another sigh, you fire up the browser console to find out what is going on and you can immediately see, standing there in all its glory: undefined is not a function
or cannot read property ‘map’ of undefined
. “Thank you, JavaScript”, you think and in despair you go back to your IDE of choice to fix the issue.
Does your work really have to look like this? It turns out it doesn’t, and we will show you that it can be significantly improved by moving to TypeScript. After finishing this blog post, you will not only know what problems JavaScript has in 2019 but also understand which of those are addressed by TypeScript and how easy it is to start using TypeScript in your projects — so easy you can even start today. All opinions are based on our experience with TypeScript at Bright IT. We work with enterprise-grade, production-ready software on a daily basis and we understand “hello world” or greenfield projects have at least an order of magnitude less moving parts than most commercially developed and maintained projects. We are extremely happy to share our experience with TypeScript with you and here we go!
Life After var that = this;
There is no denying that JavaScript has made a huge step forward thanks to the work of ECMA on development and standardisation of the JavaScript features. Modern JavaScript does not look anything like early JavaScript did. Many pain-points of its older brother have successfully been resolved and a lot of new features were aimed at making the developers’ experience better, and therefore productivity has improved in recent years. But while all of that was crucial to making progress, it simply is not enough to meet the expectations that real world applications set.
From JavaScript Scripts to JavaScript Apps
For the greater part of its life, JavaScript was used to create simple scripts or snippets that you needed to copy-and-paste into your HTML in order to add a certain behaviour to already existing markup. Because those snippets were usually small, you could just skim through a few dozen lines of code to grasp the general idea how it worked or spend a few additional minutes to understand it thoroughly, if you ever wanted to.
In modern frontend development however, that is not the case anymore. Those simple scripts have evolved into fully-blown applications, especially with newer frameworks and libraries which aid the process, making it easier than ever overall. Single Page Applications (SPAs) are the best example of making use of such tools. SPAs are—simply put—web applications. Web applications written (potentially) entirely in JavaScript. They have their own routing, state, persistence layer and ways of communicating with other services. And for certain use cases, they are absolutely fantastic.
The “Script” Part Problem
Vanilla JavaScript has an obvious scaling problem. It simply is not suited for proper application development. Applications, contrary to scripts, cannot be developed in a WORN way (write once, read never). They are typically maintained for months or even years by multiple people or even multiple development teams. Unfortunately JavaScript itself does not address any of the problems such a workflow introduces. Do you see the problem already?
Because of this clash between what JavaScript was made to do versus what it is used for today, it is eventually impossible to maintain even a mid-sized JavaScript application without (at least occasional) pain and struggle. If you fear to move files around, change their name or restructure a directory layout, you know what I’m talking about. If you get shivers even thinking about refactoring a given core functionality, you know PRECISELY what I’m on to. Something must be inherently wrong with your setup if you cannot easily change an order or number of arguments of your function.
BetterJavaScript™
The design goals of TypeScript clearly demonstrate the line of thinking behind it. The intention of TypeScript is not to create yet another, new language that looks similar to JavaScript but to enhance JavaScript with types—in a way that it stays in line with how JavaScript works.
Purists may say that it forces TypeScript to be built on unideal foundations—which is a valid point. JavaScript does not have a reputation for having the best design among other programming languages and it’s hard to argue against that in principle. On the other, maybe more pragmatic hand, following the path TypeScript has taken, allows us to mitigate quite a few real world issues without introducing new ones. Clearly however, it also constrains how powerful TypeScript can ever become. Yet, striving to find some Holy Grail while struggling with the day-to-day silly problems of JavaScript is not the most productive thing to do, is it? Don’t get me wrong, ScalaJS, ReasonML or WebAssembly are great and they certainly push the web forward—but they are not as approachable as TypeScript or even remotely as usable in existing ecosystems as is TypeScript.
The Case for Types(cript)
TypeScript solves many of the problems JavaScript comes with, yet most notably it solves the seemingly fundamental task of maintaining an application. Even with relaxed configuration, the TypeScript compiler will complain, or rather report the work it has done for you in the meantime—about issues such as calling a function with the wrong number of arguments (yes, Javascript will not, by design) or assigning a string to a number (implicit conversions are frowned upon even in strongly typed languages as Scala and you don’t ever want to add 1 to “a glass full of water”).
If you feel comfortable with the feedback TypeScript gives you—and I’m pretty sure you will, and that rather sooner than later—you can easily opt into additional features. Having more features however means TypeScript will be more strict when evaluating the correctness of your code. The compiler will not let certain classes of errors slip through upon compilation. That means you may also be warned in case a value you expect to be there is not present (at all times) or that you have forgotten to handle one of the cases in your code.
All of these examples are not just imaginary, artificial problems. They are so real that each and every JavaScript developer faces them many times and every day. Why not let TypeScript do some of the work for us instead?
Fit into the Ecosystem
You probably have hundreds if not thousands of JavaScript files that you cannot possibly convert to TypeScript overnight—and you don’t have to. TypeScript can be incrementally adopted and that approach really works. Of course, you would ideally start with all the goods TypeScript comes with, but in real-life projects it usually is not viable to stop bug fixing and new features development all of a sudden only because you intend to migrate all your existing code.
And then of course, it’s not only about having JavaScript and TypeScript files work alongside in the same project. The JavaScript ecosystem is immense in size, the number of libraries and tools is huge, and you would hardly want to abandon all of that just because the language itself is better. That is yet another point where TypeScript scores as it is designed to fit into the entire ecosystem, and not to play against it. Webpack, Babel, npm, React or Vue.js—or whatever else im that direction comes to mind. TypeScript even makes working with all of those better, and that is due to additional typings available that—if anything—will make autocompletion in your IDE finally also work in the Frontend.
Less Unnecessary IDE-to-browser-and-back-again Journeys
Frontend development is not about the functionality—it is mostly about the user. Of course, it is the functionality that matters the most to business and without it, a website is merely an online catalogue or brochure. But because so many requirements or expectations—either from users or business—are non-functional, it is hard to test those without having a look in the web browser—ideally having a live preview at all times. We have given up our attempts to write tests for “the design looks good” or “animations are slick and smooth” quite some time ago.
Tight and fast feedback loops between code and preview is absolutely crucial for successful state-of-the-art frontend delivery. But you know what? That can be more efficient. With TypeScript, you can stay focused on the code for longer because its compiler will ask you to fix issue it found automatically BEFORE you ever need to check them manually in the browser. Isn’t that great?
And You Are Only One Step Away...
My favourite part of TypeScript, that really sets it apart from other proposals for improvements on top of JavaScript for frontend development, is that you are most probably just one small step away from using in. Yes, using it at work, not in your hello world pet project.
Especially after Babel 7 introduced first-class support for TypeScript with @babel/preset-typescript
, you can just include it in your existing build chain, change the extension of an arbitrary file from “.js” to “.ts”, add the least restrictive “tsconfig.json” (ensure you have “allowJs”: true there) and are you are good to go. You just started using TypeScript!
Bright IT + TypeScript = <3
If this article sounds like an advertisement for TypeScript… we have to admit it kind of is. It’s not a sponsored post though (by the way, TypeScript is 100% open source, backed by Microsoft)—it simply is candid advice on how we believe things should be done, and why.
We started using TypeScript in 2017 and we have loved it every since. By now it is our default choice for all frontend development hands down. The IDE support is great, toolchain JustWorks™ and we have had absolutely no real issues with it so far (nothing that wasn’t easily solved with one Google search). The benefits that TypeScript brings are immense—once you have experienced it, you get a feeling that something has inherently been missing in JavaScript.
We encourage you to give TypeScript a try and bring your frontend development to the next level. And as always we are happy to help your organisation to jump onto the right track. If you have any more questions or doubts, don’t hesitate to reach out to us.
And you know what? Our colleagues who mainly work with Scala are now much more keen on jumping onto frontend codebase and to start contributing. Isn’t that a sign that there’s something more about TypeScript? Try it for yourself. Undefined is not a function
, never again.